mirror of
https://github.com/saltstack/salt.git
synced 2025-04-17 10:10:20 +00:00
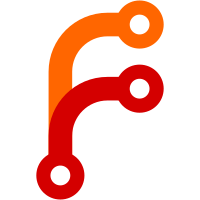
* fixes saltstack/salt#62372 unable to use random shuffle and sample functions as Jinja filters * move random_shuffle and random_sample logic to utils * static seed in tests seems to have shifted * static seed in tests require hash module * Change Tiamat to onedir in release notes * Reinstate known issues * Update release notes with onedir package support policy * need to check the version of Netmiko python library and then import the exceptions from different locations depending on the result. * Adding changelog. * swap out if...else for double try...except. * Remove extra fix we don't need anymore * [Docs] include onedir system python note * Update all platforms to use pycparser 2.21 or greater for Py 3.9 or higher, fixes fips fault with openssl v3.x * Remove the PyObjC dependency Signed-off-by: Pedro Algarvio <palgarvio@vmware.com> * Add "<tiamat> python" subcommand to allow execution or arbitrary scripts via bundled Python runtime * Document usage of bundled Python runtime for Client API * Use explicit locals for custom script execution, handle exception in similar fashion as Python * Remove old __file__ replacement * Apply suggestions from code review Co-authored-by: Pedro Algarvio <pedro@algarvio.me> Co-authored-by: nicholasmhughes <nicholasmhughes@gmail.com> Co-authored-by: Alyssa Rock <alyssa.rock@gmail.com> Co-authored-by: Gareth J. Greenaway <gareth@saltstack.com> Co-authored-by: Twangboy <leesh@vmware.com> Co-authored-by: David Murphy < dmurphy@saltstack.com> Co-authored-by: Pedro Algarvio <palgarvio@vmware.com> Co-authored-by: Lukas Raska <lukas@raska.me> Co-authored-by: Pedro Algarvio <pedro@algarvio.me>
82 lines
1.9 KiB
Python
82 lines
1.9 KiB
Python
"""
|
|
:codeauthor: Rupesh Tare <rupesht@saltstack.com>
|
|
"""
|
|
|
|
import re
|
|
|
|
import pytest
|
|
|
|
import salt.modules.mod_random as mod_random
|
|
import salt.utils.pycrypto
|
|
from salt.exceptions import SaltException, SaltInvocationError
|
|
from tests.support.mock import patch
|
|
|
|
|
|
def _test_hashlib():
|
|
try:
|
|
import hashlib
|
|
except ImportError:
|
|
return False
|
|
|
|
if not hasattr(hashlib, "algorithms_guaranteed"):
|
|
return False
|
|
else:
|
|
return True
|
|
|
|
|
|
SUPPORTED_HASHLIB = _test_hashlib()
|
|
|
|
pytestmark = [
|
|
pytest.mark.skipif(
|
|
SUPPORTED_HASHLIB is False,
|
|
reason="Hashlib does not contain needed functionality",
|
|
),
|
|
]
|
|
|
|
|
|
@pytest.fixture
|
|
def configure_loader_modules():
|
|
return {mod_random: {}}
|
|
|
|
|
|
def test_hash():
|
|
"""
|
|
Test for Encodes a value with the specified encoder.
|
|
"""
|
|
assert mod_random.hash("value")[0:4] == "ec2c"
|
|
pytest.raises(SaltException, mod_random.hash, "value", "algorithm")
|
|
|
|
|
|
def test_str_encode():
|
|
"""
|
|
Test for The value to be encoded.
|
|
"""
|
|
pytest.raises(SaltInvocationError, mod_random.str_encode, "None", "abc")
|
|
pytest.raises(SaltInvocationError, mod_random.str_encode, None)
|
|
assert mod_random.str_encode("A") == "QQ=="
|
|
|
|
|
|
def test_get_str():
|
|
"""
|
|
Test for Returns a random string of the specified length.
|
|
"""
|
|
assert mod_random.get_str(length=1, chars="A") == "A"
|
|
assert len(mod_random.get_str(length=64)) == 64
|
|
ret = mod_random.get_str(
|
|
length=1,
|
|
lowercase=False,
|
|
uppercase=False,
|
|
printable=False,
|
|
whitespace=False,
|
|
punctuation=False,
|
|
)
|
|
assert not re.search(r"^[a-zA-Z]+$", ret), "Found invalid characters"
|
|
assert re.search(r"^[0-9]+$", ret), "Not found required characters"
|
|
|
|
|
|
def test_shadow_hash():
|
|
"""
|
|
Test for Generates a salted hash suitable for /etc/shadow.
|
|
"""
|
|
with patch.object(salt.utils.pycrypto, "gen_hash", return_value="A"):
|
|
assert mod_random.shadow_hash() == "A"
|